在Android中使用 asmack 实现文件的接收和发送 - 移动开发
本篇主要是基于最近帮助朋友在 Android 中使用 asmack 库实现文件的接收和发送 功能时,写了个参考示例,这里做个记录,以便于自己以后参考。
文件传输相关的XEP协议参考:
2【XEP-0095】StreamInitiation: http://xmpp.org/extensions/xep-0095.html
2【XEP-0096】SI FileTransfer: http://xmpp.org/extensions/xep-0096.html
2【XEP-0030】ServiceDiscovery: http://xmpp.org/extensions/xep-0030.html
2【XEP-0065】SOCKS5Bytestreams: http://xmpp.org/extensions/xep-0065.html
2【XEP-0066】Out of BandData: http://xmpp.org/extensions/xep-0066.html
2【XEP-0047】In-BandBytestreams: http://xmpp.org/extensions/xep-0047.html
本实例中主要采用的是SI + IBB 的方式发送和接收文件。
1,首先在连接和登录之前设置 ProviderManager,同时设置好全局的文件传输管理器(FileTransferManager) fileTransferManager,如下:
/** ProviderManager pm = ProviderManager.getInstance(); // Private Data Storage // Roster Exchange // Message Events // Chat State // XHTML // Group Chat Invitations // Service Discovery # Items // Service Discovery # Info // Data Forms // MUC User // MUC Admin // MUC Owner // Delayed Delivery // Version // VCard // Offline Message Requests // Offline Message Indicator // Last Activity // User Search // SharedGroupsInfo // JEP-33: Extended Stanza Addressing // FileTransfer // Privacy pm.addIQProvider(”command”, ”http://jabber.org/protocol/commands”, pm.addExtensionProvider(”malformed-action”, pm.addExtensionProvider(”bad-locale”, pm.addExtensionProvider(”bad-payload”, pm.addExtensionProvider(”bad-sessionid”, pm.addExtensionProvider(”session-expired”, try fileTransferManager = new FileTransferManager(connection); |
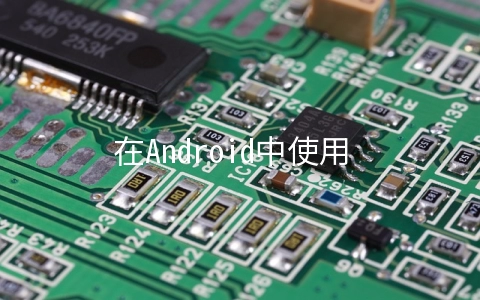
2,发送文件,如下:
Button btnSendFile = (Button) rootView.findViewById(R.id.send_file_btn); // 将发送文件的操作采用 AsyncTask 的方式实现(SendFileTask): @Override if (params.length < 1) { Uri uriFile = params[0]; return Long.valueOf(0); |
3,接收文件,主要通过设置 文件传输监听器 来实现:
FileTransferManager ftm = MainHelloIM.getInstance().getFileTransferManager(); |
下面为完整的文件传输的消息交互流程:
//—– negotiation profile and stream hhy -> blue blue -> hhy //—– service discovery hhy -> blue blue -> hhy hhy -> [server] blue -> hhy [server] -> hhy hhy -> [server proxy] [server proxy] -> hhy hhy -> [server pubsub] [server pubsub] -> hhy hhy -> [server search] [server search] -> hhy hhy -> [server conference] [server conference] -> hhy //—– 首先是采用 bytestreams 方式传输文件的 hhy -> [server proxy] [server proxy] -> hhy hhy -> blue blue -> hhy //—– 上面方式不行,采用 IBB 方式传输 hhy -> blue blue -> hhy hhy -> blue blue -> hhy hhy -> blue blue -> hhy |
发布于:2023-02-10,除非注明,否则均为
原创文章,转载请注明出处。
发表评论